Taking the Typescript Challenge
A fantastic repository that was shown to me about a month ago is the Type Challenges repository - a collection of Typescript puzzles made to help understand the different abilities and powers of Typescript. I think they say it best:
This project is aimed at helping you better understand how the type system works, writing your own utilities, or just having fun with the challenges.
Type Challenge Team
How to play
You can play it in a few ways but I think the two best options are:
- In Typescript Playground online.
- In Visual Studio Code through the excellent extension.
VS Code
My favourite way to play this is in VS Code with the extension. It comes with a few advantages over the other options. It has all the challenges in order by difficulties, tags and authors so you can easily pick what works best for you.
When you select a challenge it gives you a description of what you need to do, gives you a list of related challenges, and gives you a link to solutions if you require.
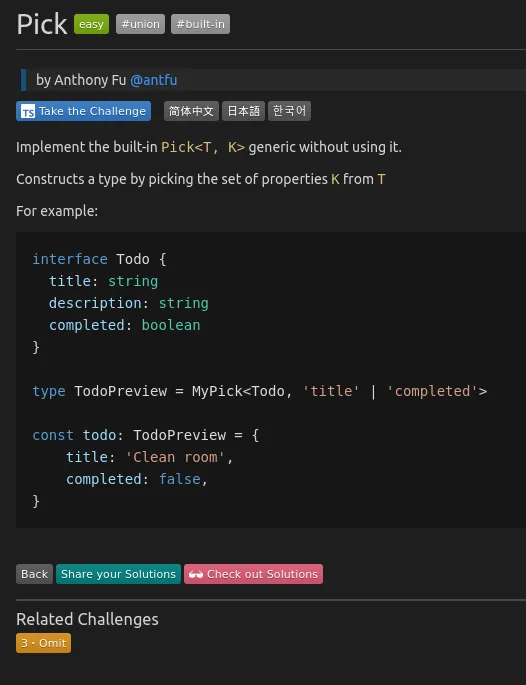
Format
The tests themselves are arranged in a specific format, utilising a couple of custom types to verify that your answer is correct.
One of the core ones is the Expect
type. It is a generic type that expects the type passed in to equal true
. If the passed in type is not true you will get a type error.
Next there are a range of comparator types that can compare two different types and resolve to a type that is true
or false
. For instance, Equal
is used to check that the two passed in types are the same.
Equal<string, string> // Resolves to true
Equal<string, boolean> // Resolves to false
This is cool as it isn’t running your code, but just verifying your types.
My Completions
Over time I will be filling out my attempts at some of these solutions. They can be seen below:
- Pick
- Readonly
- Tuple to Object
- First of Array
- Length of A Tuple
- Exclude
- Awaited
- If
- Array Concatenation
- Includes
- Push And Unshift
- Parameters And Return Type
Syntax Learnt
Over time I will update the list as I come across new syntax.
{ [Key in keyof T]: T[Key] }
- Object with keys and values from T
{ readonly key: type }
- Set the key to be read only
{ [Value in T[number]: Value }
- Get the value of an array element at T[number]
What to read more? Check out more posts below!