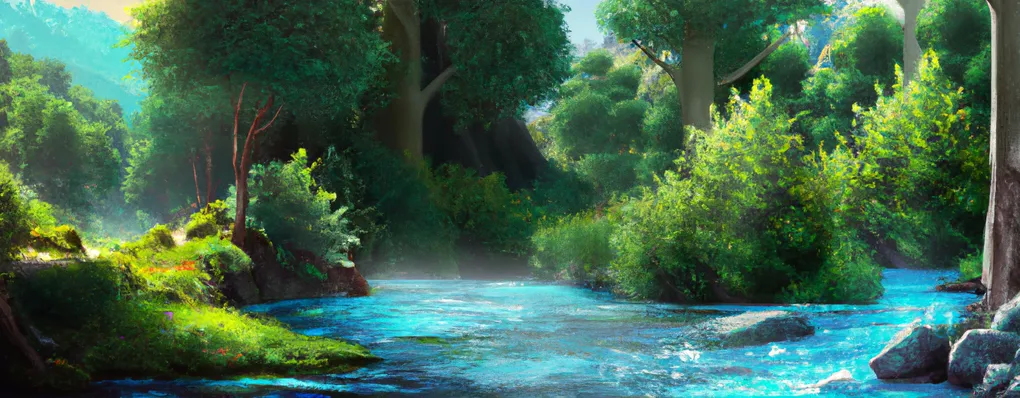
Pure Functions
What are Pure Function?
Pure functions are any function that will always return the same result for a given input, and does not read or write data outside its scope.
This can be any interaction, from reading external variables, making API requests or even just using console.log
.
In the above example, our impure version of the function is logging out the two parameters. You may argue that this isn’t really hitting anything external but you can never guarantee that console.log
is actually running what you expect. Many logging programs overwrite the existing log function to do additional things.
The Pros
- Pure Functions are much easier to test - You do not have to create stubs for any functions it may call as it relies 100% on the inputs you give it.
- Pure Functions are easier to debug - for the same reasons it is easier to test.
- Parallelisation - The function can be called concurrently as it has no side effects.
The Cons
The core issue with pure functions is that you have to pass them everything they need, and you cannot mutate state within them. This means they cannot be used everywhere, and sometime juggling all the parameters can be a little messy.
But we need side effects!
It is true that any program you create is likely to require many side effects for it to be of any use. Almost every interaction requires some form of side effect, including but not limited to:
- API requests
- Querying the DOM - User Input, Finding Elements etc.
- Changing or Viewing any state - Local Storage, Variables, Databases
Given this, you may wonder how pure functions can be useful. It comes down to the idea that the most likely place for a problem is somewhere we either read or write to a location we don’t fully control within our current scope.
We do have control though!
It may or may not be true that you have control now, but you will never remember every bit of code you write and at some point the control you thought you had is ruined by a misunderstanding, a mistake or another person entirely.
There are also lots of things out of our control at least some of the time such as sharing parts of the code with other teams, 3rd party tools, internet connections and server up time.
It is always best to narrow the locations that can have these issues as you never know who else will be working on what you are writing now.
How to write
Creating pure functions is super easy! A good rule is that if you pass a function what it needs and don’t reference any other code it is pure.
function firstLetterUpperCase(str) {
const [first, ...rest] = str;
return first.toUpperCase() + rest.join('');
}
firstLetterUpperCase("string");
See how everything the firstLetterUpperCase
function needed was provided by the parameter. There are inbuilt functions used but if we just use pure ones, there is no issue! Everything is nice and contained within our function.
This code can now be used anywhere because it is pure. No external dependencies to be brought over, no state that needs to exist already. It is as simple as a function can get.
A more complex example
We are building up some foundations now. More complex examples will be shown in the sections for Currying
and Composition
.
What to read more? Check out more posts below!